The ImageKit10 VCL allows you to process image effects in a
variety of useful ways. One of the most useful ways to involves the
use of a mask image. A mask image is simply a black and white
image. When a mask image is layered on the original image, the mask
determines what portion of the original image will be processed.
When using the ImageKit.Effect methods (or the IKEffect.dll user
functions), an image and mask image are created. Below are a few
examples of how to process image effects with both the ImageKit10
VCL and ImageKit10 DLL versions:
Selecting images (SelectImage)
In the ImageKit10 VCL, there are three ways in which images or
portions of images can be selected for processing:
1 - selecting the total image
2 - selecting a polygon
3 - selecting an ellipse
In all cases, the image and the mask image are generated and, when
using the ImageKit10 VCL controls, the results are returned in the
ImageHandle
property or the
ImageKit.Layer(LayerNo).ImageHandle property. (When the
ImageKit10 Dll functions are used, the results are returned in the
IKSELECT_IMAGE structure).
Below is a brief explanation about each of these and the images and
mask images that they generate.
* When you select the whole image, an all white mask image is
generated. If the mask image is not needed, please free it it.
[Delphi Example (VCL)]
VImageKit1.LayerNo :=
-1; //process the base
image
VImageKit1.ImageHandle :=
ImgHandle; //set the
image
VImageKit1.Effect.MaskImageHandle :=
0; //free the mask
image handle
VImageKit1.Effect.SelectMode
:= vikEffectAll; //process the whole image
Ret :=
VImageKit1.Effect.SelectImage(255,0,0); //execute the SelectImage
method
[Delphi Example (DLL)]
// the following function
creates the image and the mask image
IKSelectImageEx(ImgHandle,
DstHandle, 1, pt(0), 0, rc, True, 255, 0, 0, "Select", "Select",
"Cancel");
IKFreeMemory(ImgHandle); // Deletes the input image handle.
Note: If the input image handle is not deleted, the image will
remain in the memory.
Selecting a polygonal area in the image (SelectMode = 2)
Polygons can be selected by passing the coordinate points of the
polygon as the arguments. The size of the output image and mask
image is the size of the smallest rectangle that can circumscribe
the polygon.
[Delphi Example (VCL)]
Pt: array[0..2] of
TPoint;
Pt[0].X := 90; Pt[0].Y :=
60; //Sets the
coordinates of the points of the polygon
Pt[1].X := 560; Pt[1].Y :=
100;
Pt[2].X := 410; Pt[2].Y :=
390;
VImageKit1.LayerNo :=
-1; //process the base
image
VImageKit1.ImageHandle :=
ImgHandle; //set the
image
VImageKit1.Effect.MaskImageHandle :=
0; //free the mask
image handle
VImageKit1.Effect.InOut :=
vikInside; //process
inside the polygon
VImageKit1.Effect.SelectMode
:= vikEffectPolygon; //process the polygonal area on the
image
Ret =
VImageKit1.Effect.SelectImage(Pt, 255, 0, 0); //execute SelectImage method
[Delphi Example (DLL)]
Pt: array[0..2] of
TPoint;
Pt[0].X := 90; Pt[0].Y :=
60; // Sets the
coordinates of the points of the polygon
Pt[1].X := 560; Pt[1].Y :=
100;
Pt[2].X := 410; Pt[2].Y :=
390;
// the following function
creates the image and the mask image
IKSelectImageEx(ImgHandle,
DstHandle, 2, Pt(0), 3, rc, True, 255, 0, 0, 0, "Select", "Select",
"Cancel")
IKFreeMemory(ImgHandle);
// Deletes the effect control
input image handle
ImgHandle := 0;
Note: If the input image handle is not deleted, the image will
remain in the memory.
Selecting an elliptical area in the image (SelectMode =
3)
An ellipse can be selected by setting the location of the
coordinate points for the bounding rectangle in the RectLeft,
RectTop, RectBottom, and RectRight properties (when using the
ImageKit10 VCL controls) or by setting these coordinates in the
RECT structure (when using the DLL functions). The size of the
output image and mask image is the size of the smallest bounding
rectangle that circumscribes the ellipse.
[Delphi Example (VCL)]
VImageKit1.Effect.RectLeft :=
100;
VImageKit1.Effect.RectTop := 50;
VImageKit1.Effect.RectRight := 540;
VImageKit1.Effect.RectBottom := 400;
VImageKit1.LayerNo := -1;
VImageKit1.ImageHandle := ImgHandle;
VImageKit1.Effect.MaskImageHandle := 0;
VImageKit1.Effect.InOut := vikInside;
VImageKit1.Effect.SelectMode :=
vikEffectEllipse;
Ret :=
VImageKit1.Effect.SelectImage(255,0,0);
[Delphi Example (DLL)]
rc: TRect;
Rect.Left :=
100; Rect.Top := 50;
Rect.Right :=
540; RectBottom := 400;
IKSelectImageEx(ImgHandle,
DstHandle, 3, pt(0), 0, rc, True, 255, 0, 0, 0, "Select", "Select",
"Cancel");
IKFreeMemory(ImgHandle);
ImgHandle := 0;
Embossing images (Emboss)oss)
In the following, we have choosen to use the Emboss method as an
example of applying an effect.
Using an existing mask image (SelectMode = 0)
By setting the SelectMode to 0, an existing mask image can be used
to determine the area on the original image that will be processed.
The input mask image, input image, and the output image are shown
below. The ImgHandle can refer to an image that is 1, 4, 8, 16, 24,
or 32 bit color, however for the Emboss method, the input image
must be 8G, 16, 24, or 32 bit. The MskHandle refers to a black and
white 1 bit color image, where the white portion of the mask
determines the area on the image that will be processed and the
black portion determines the area that will be masked.
[Delphi Example (VCL)]
VImageKit1.LayerNo := -1;
VImageKit1.ImageHandle := ImgHandle;
VImageKit1.Effect.MaskImageHandle :=
MskHandle;
VImageKit1.Effect.SelectMode :=
vikEffectMask;
Ret :=
VImageKit1.Effect.Emboss(0,3,128)
[Delphi Example (DLL)]
Src: IKSELECT_IMAGE;
Src.hImgBmh := ImgHandle;
Src.hMskBmh := MskHandle;
// executes the Emboss
functiontion
DstHandle := IKEmboss(Src, 0,
pt(0), 0, rc, True, 0, 3, 128, 0, "Emboss", " Emboss ",
"Cancel");
IKFreeMemory(ImgHandle); // Deletes the input image handle and input mask
handle
IKFreeMemory(MskHandle); // If these are not deleted they will remain in the
memory.
ImgHandle := 0;
MskHandle := 0;
[Delphi Example (VCL)]
VImageKit1.LayerNo := -1;
VImageKit1.ImageHandle := ImgHandle;
VImageKit1.Effect.MaskImageHandle := 0;
VImageKit1.Effect.SelectMode :=
vikEffectAll;
Ret :=
VImageKit1.Effect.Emboss(0,3,128);
[Delphi Example (DLL)]
Src: IKSELECT_IMAGE;
Src.hImgBmh := ImgHandle;
Src.hMskBmh := 0;
// executes the Emboss
functionunction
DstHandle := IKEmboss(Src, 1,
pt(0), 0, rc, True, 0, 3, 128, 0, "Emboss", "Emboss",
"Cancel");
IKFreeMemory(ImgHandle); // Deletes the input image handle. If not freed the
image data will remain in the memory.memory.
ImgHandle := 0
*Note: When the InOut property is TRUE, the area inside the polygon
is embossed. When the InOut property is False, the area outside the
polygon is processed.cessed.
[Delphi Example (VCL)]
Pt: array[0..2] of
TPoint;
Pt[0].X := 90; Pt[0].Y :=
60; // Sets the coordinates of the
points of the polygon
Pt[1].X := 560; Pt[1].Y :=
100;
Pt[2].X := 410; Pt[2].Y :=
390;
VImageKit1.LayerNo := -1;
VImageKit1.ImageHandle := ImgHandle;
VImageKit1.Effect.MaskImageHandle := 0;
VImageKit1.Effect.InOut := vikInside;
VImageKit1.Effect.SelectMode :=
vikEffectPolygon;
Ret := VImageKit1.Effect.Emboss(Pt,
0,3,128);
[Delphi Example (DLL)]
Src: As
IKSELECT_IMAGE;
Pt: array[0..2] of
TPoint;
Pt[0].X := 90; Pt[0].Y :=
60;
Pt[1].X := 560; Pt[1].Y :=
100;
Pt[2].X := 410; Pt[2].Y :=
390;
Src.hImgBmh :=
ImgHandle; // Sets the image into
the input image handle
Src.hMskBmh := 0;
// Executes the Emboss
function
DstHandle := IKEmboss(Src, 2,
pt(0), 0, rc, True, 0, 3, 128, 0, "Emboss", "Emboss",
"Cancel")
IKFreeMemory(ImgHandle); //
Deletes the input image handle.
ImgHandle := 0;
*Note: When the InOut property is TRUE, the area inside the ellipse
is embossed. When the InOut property is False, the area outside the
ellipse is processed.
[Delphi Example (VCL)]
VImageKit1.Effect.RectLeft :=
100;
VImageKit1.Effect.RectTop := 50;
VImageKit1.Effect.RectRight := 540;
VImageKit1.Effect.RectBottom := 400;
VImageKit1.LayerNo := -1;
VImageKit1.ImageHandle := ImgHandle;
VImageKit1.Effect.MaskImageHandle := 0;
VImageKit1.Effect.InOut := vikInside;
VImageKit1.Effect.SelectMode :=
vikEffectEllipse;
Ret :=
VImageKit1.Effect.Emboss(0,3,128);
[Delphi Example (DLL)]
Src:
IKSELECT_IMAGE;
rc: TRect;
Rect.Left := 100; Rect.Top :=
50;
Rect.Right := 540; Rect.Bottom
:= 400;
Src.hImgBmh :=
ImgHandle; // Sets the image into
the input image handle
Src.hMskBmh := 0;
// Executes the Emboss
function
DstHandle := IKEmboss(Src, 3,
pt(0), 0, rc, True, 0, 3, 128, 0, "Emboss", "Emboss",
"Cancel");
IKFreeMemory(ImgHandle) // Deletes the input image handle
ImgHandle := 0;
* Note: The Emboss method, like many other Effect methods and
IKEffect dll user functions, takes an image handle and a mask image
handle as input and returns an image handle as output. Below is a
list of other methods and dll functions that are used in the same
way.
Method Name (VCL) | Function Name (DLL) | Explanation |
---|---|---|
AntiAlias | IKAntiAlias | Smooths the edges in the image. |
Blur | IKBlur | Blurs the image |
Canvas | IKCanvas | Creates a canvas effect on the image |
Chroma | IKChroma | Adjusts chrominance |
CustomFilter | IKCustomFilter | Creates a user defined custom filter |
GlassTile | IKGlassTile | Creates a glass tile effect on the image |
Lens | IKLens | Creates a lens effect on the image |
Mosaic | IKMosaic | Creates a mosaic on the image |
MotionBlur | IKMotionBlur | Blurs the image as if it were in motion |
OilPaint | IKOilPaint | Creates an oil painting like effect on the image |
Outline | IKOutline | Creates an outline of the image |
RemoveNoise | IKRemoveNoise | Reduces noise in the image |
RGBGamma | IKRGBGamma | Adjusts image RGB values using gamma correction |
RGBLevel | IKRGBLevel | Increase or decreases image RGB values |
RGBRev | IKRGBRev | Reverses image RGB values |
RGBSpline | IKRGBSpline | Adjusts image RGB values using spline interpolation |
Ripple | IKRipple | Creates a ripple effect on the image |
Sharp | IKSharp | Sharpens contours |
UnifyColor | IKUnifyColor | Converts all colors within a certain range into one color |
Waves | IKWaves | Creates a wave like effect on the image |
WhirlPinch | IKWhirlPinch | An effect like pinching the image and twisting your fingers |
YCCGamma | IKYCCGamma | Adjusts image YCrCb values using gamma correction |
YCCLevel | IKYCCLevel | Increase or decreases image YCrCb values |
YCCRev | IKYCCRev | Reverses image YCrCb values |
YCCSpline | IKYCCSpline | Adjusts image YCrCb values using spline interpolation |
Pasting Images (PasteImage)
The PasteImage method (IKPasteImage function) allows you to use masks to select a portion of an image and paste this into another image. This technique shown below, involves using the MskHandle and ImgHandle then pasting those into SrcHandle. The result can be seen in the DstHandle.
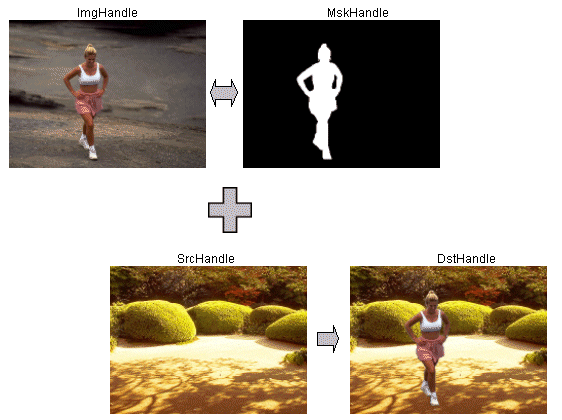
[Delphi Example (VCL)]
VImageKit1.LayerNo := -1;
VImageKit1.ImageHandle := ImgHandle;
VImageKit1.Effect.MaskImageHandle := MskHandle;
Ret := VImageKit1.Effect.PasteImage(SrcHandle,0,False,False,255,False,0,0,0,255,255,255,192,144,False);
if Ret = False then Exit;
VImageKit1.FreeMemory(SrcHandle);
SrcHandle := 0;
[Delphi Example (DLL)]
Src: IKSELECT_IMAGE;
Src.hImgBmh := ImgHandle;
Src.hMskBmh := MskHandle;
DstHandle := IKPasteImage(SrcHandle, Src, 0, FALSE, FALSE, 255, FALSE, 0, 0, 0, 255, 255, 255 192, 144, FALSE, 0, "Paste", "Paste", "Cancel");
IKFreeMemory(SrcHandle);
IKFreeMemory(Src.hImgBmh);
IKFreeMemory(Src.hMskBmh;)
SrcHandle := 0;
Src.hImgBmh := 0;
Src.hMskBmh := 0;
Layering Images (LayerImage)
The LayerImage method (IKLayer) is another way two images are combined. This technique involves setting a specific color to transparent and layering two images.
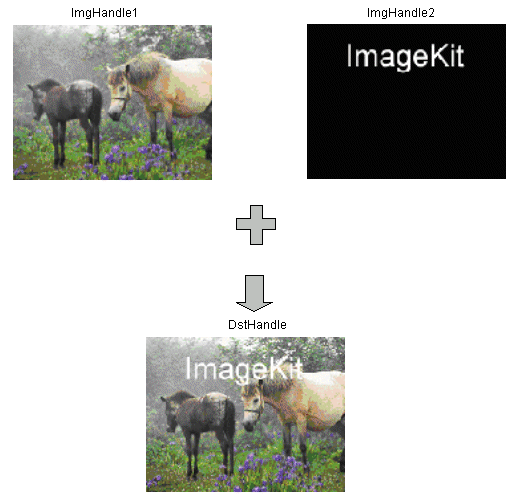
[Delphi Example (VCL)]
VImageKit1.LayerNo := -1;
Ret := VImageKit1.Effect.LayerImage(ImgHandle1, ImgHandle2,255,True,0,0,0,255,255,255,0,0,False);
if Ret = False Then Exit;
VImageKit1.FreeMemory(ImgHandle1);
ImgHandle1 := 0;
VImageKit1.FreeMemory(ImgHandle2);
ImgHandle2 := 0;
[Delphi Example (DLL)]
DstHandle: THandle;
DstHandle := IKLayer(ImgHandle1, ImgHandle2, 255, True, 0, 0, 0, 255, 255, 255, 0, 0, False, 0, "Layer", "Layer", "Cancel");// Executes the layer function
IKFreeMemory(ImgHandle1)
IKFreeMemory(ImgHandle2)
ImgHandle1 = 0
ImgHandle2 = 0
Resizing Images (Resize)
When using the Resize method (or IKResize) both images and mask image are resized together
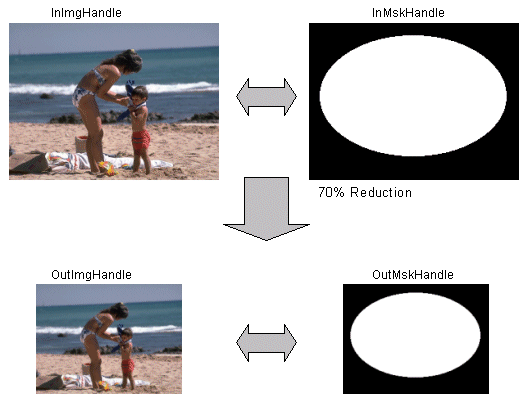
[Delphi Example (VCL)]
VImageKit1.LayerNo := -1;
VImageKit1.ImageHandle := ImgHandle;
VImageKit1.Effect.MaskImageHandle := MskHandle;
Ret := VImageKit1.Effect.Resize(179, 134, 1);
[Delphi Example (DLL)]
Src: IKSELECT_IMAGE;
Src.hImgBmh := InImgHandle;
Src.hMskBmh := InMskHandle;
IKResizeEx(Src, Dst, 179, 134, True, 0, "Reduction", "Reduction", "Cancel")
IKFreeMemory(InImgHandle);
IKFreeMemory(InMskHandle);
InImgHandle := 0;
InMskHandle := 0;
*Note: The Resize method (IKResize function) takes an image and mask image as input and returns an image and mask image as output. The following methods (dll functions) are used in the same way.
Method Name (VCL) | Function Name (DLL) | Explanation |
---|---|---|
Rotation | IKRotationEx | Rotates an image and mask image |